I have a SharePoint Designer 2013 workflow which sometimes ends up in a Suspended state with the following cryptic HTTP 400 error:
RequestorId: af5849b3-e5e4-5875-0000-000000000000. Details: RequestorId: af5849b3-e5e4-5875-0000-000000000000. Details: An unhandled exception occurred during the execution of the workflow instance. Exception details: System.ApplicationException: HTTP 400 {“Transfer-Encoding”:[“chunked”],”X-SharePointHealthScore”:[“0″],”SPClientServiceRequestDuration”:[“44″],”SPRequestGuid”:[“af5849b3-e5e4-5875-bc88-32fb13dd46f3″],”request-id”:[“af5849b3-e5e4-5875-bc88-32fb13dd46f3″],”X-FRAME-OPTIONS”:[“SAMEORIGIN”],”MicrosoftSharePointTeamServices”:[“15.0.0.4481″],”X-Content-Type-Options”:[“nosniff”],”X-MS-InvokeApp”:[“1; RequireReadOnly”],”Cache-Control”:[“max-age=0, private”],”Date”:[“Tue, 29 Apr 2014 22:21:08 GMT”],”Server”:[“Microsoft-IIS\/7.5″],”X-AspNet-Version”:[“4.0.30319″],”X-Powered-By”:[“ASP.NET”]} at Microsoft.Activities.Hosting.Runtime.Subroutine.SubroutineChild.Execute(CodeActivityContext context) at System.Activities.CodeActivity.InternalExecute(ActivityInstance instance, ActivityExecutor executor, BookmarkManager bookmarkManager) at System.Activities.Runtime.ActivityExecutor.ExecuteActivityWorkItem.ExecuteBody(ActivityExecutor executor, BookmarkManager bookmarkManager, Location resultLocation)
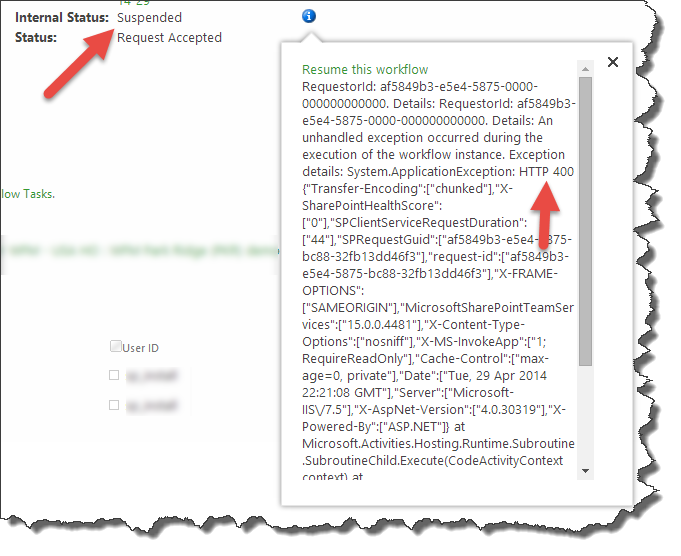
I knew this was happening on a “Send an email” action in the workflow, but was having some trouble tracking down the issue and couldn’t find anything obviously wrong with the way things were setup.
After increasing the diagnostic logging levels I finally came across the following error in the ULS log:
Exception occured in scope Microsoft.SharePoint.Utilities.SPUtility.SendEmail. Exception=System.ArgumentException: The specified string is not in the form required for a subject.
at System.Net.Mail.Message.set_Subject(String value)
at Microsoft.SharePoint.Utilities.SPUtility.SendEmail_Client(EmailProperties properties)
at Microsoft.SharePoint.ServerStub.Utilities.SPUtilityServerStub.InvokeStaticMethod(String methodName, ClientValueCollection xmlargs, ProxyContext proxyContext, Boolean& isVoid)
at Microsoft.SharePoint.Client.ServerStub.InvokeStaticMethodWithMonitoredScope(String methodName, ClientValueCollection args, ProxyContext proxyContext, Boolean& isVoid)
The email being sent out used the customer name in the subject line.
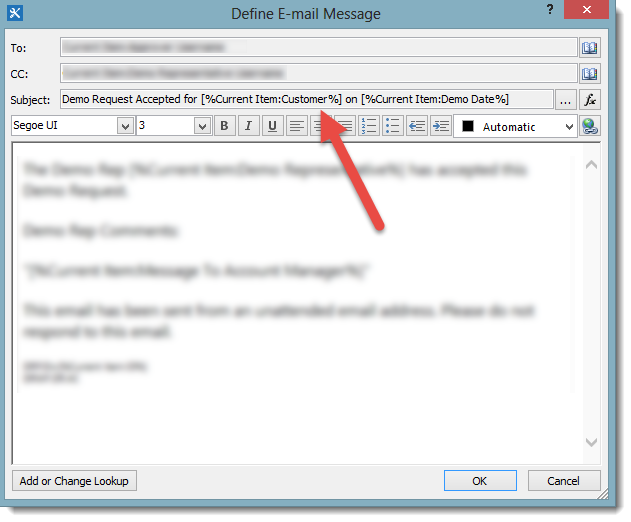
In this particular instance the customer name was coming from an InfoPath form and when I looked in the form I found a carriage return and line feed at the end of the customer name which was causing the error.

I fixed the source data which was causing the problem, but I still had forms which included the bad data. I thought if I could strip out the carriage return and line feed in the workflow before sending the email that would solve my problem.
Unfortunately there is no built-in workflow actions to do this kind of string replacement with special characters. So I even went to the trouble of trying to create my own custom SharePoint 2103 workflow action to do this, thinking that it would be reusable.

Setting ResultString to SourceString.Replace(“\r\n”, “”)
The custom action stubbornly refuses to work with escaped characters. I tried every variation of this I could imagine even using characters escaped for Regex expressions, as under the hood the workflow engine is using Regex to do the replacement. The custom action works beautifully if you use a regular string replacement like SourceString.Replace(“abc”, “”), but special characters it does not like. I finally gave up with this approach and fixed the bad data in the form before the workflow got fired.